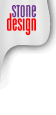 |
TOC | PREV | NEXT
TableViews
GIFfun displays information about each of its gifs in an NSTableView subclass, SDTableView. Loading a TableView is easy, especially if you have an array, gifFileArray, of "GifInfo"s, a class which holds the information of each file: its path, its image and its associated delay:
@interface GifInfo : NSObject
{
unsigned int delay;
NSString *path;
NSImage *image;
}
...
- (NSString *)path;
- (NSImage *)image;
...
In GifController.m, our tableView's dataSource (the object which will provide the data for the table after "reloadData" message is sent to the tableView), we load the table through the delegate methods that follow. One point of interest is the column's "identifier" - this is a string which you enter in the InterfaceBuilder Inspector when you double-click the header cell of a column in a tableview. Here we define our identifiers that we use in IB:
// IB TableView Identifiers:
#define I_NUMBER @"number"
#define I_PATH @"gifPath"
#define I_DELAY @"delay"
#define I_IMAGE @"image"
#define IMAGE_COLUMN_NUMBER 2
Also to note, to add a column in IB, select a column, copy and paste.
//
// NSTableView dataSource delegate methods:
//
- (int)numberOfRowsInTableView:(NSTableView *)tv
{
return [gifFileArray count];
}
- tableView:(NSTableView *)tv
objectValueForTableColumn:(NSTableColumn *)tc
row:(int)row
{
NSString *ident = [tc identifier];
if (tv == tableView) {
if ([ident isEqual:I_NUMBER]) return [NSNumber numberWithInt:row + 1];
else if ([ident isEqual:I_IMAGE])
return [[gifFileArray objectAtIndex:row]image];
else if ([ident isEqual:I_PATH])
return [self uiPath:[gifFileArray objectAtIndex:row]];
else if ([ident isEqual:I_DELAY])return [NSNumber numberWithInt:[[gifFileArray objectAtIndex:row]delay]];
}
return @"";
}
- (void)tableView:(NSTableView *)tv setObjectValue:(id)object forTableColumn:(NSTableColumn *)tc row:(int)row;
{
NSString *ident = [tc identifier];
if (tv == tableView) {
if ([ident isEqual:I_DELAY])
[[gifFileArray objectAtIndex:row]setDelay:[object intValue]];
}
}
TOC | PREV | NEXT
Created by Stone Design's Create on 4/30/1998
|
|